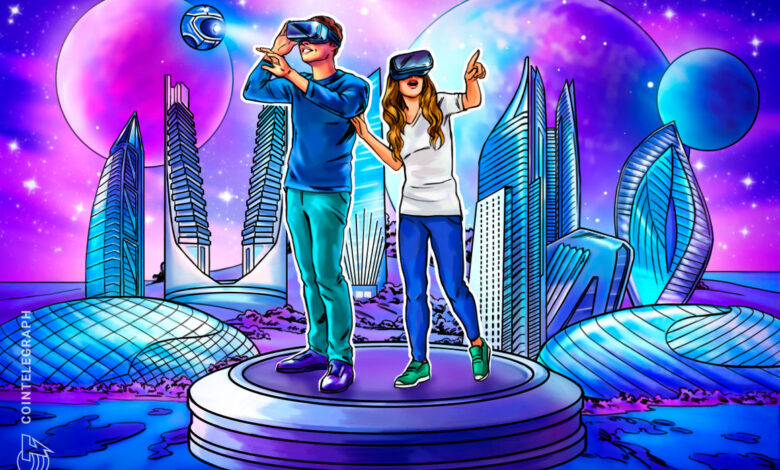
GameFi could be the answer to unemployment for some — Aussie game studio
[ad_1]
Australian-based Web3 game studio Ninja Syndicate’s CEO and founder believes GameFi could usher in a new era where users can earn a living wage through blockchain games.
Speaking to Cointelegraph, founder John Nguyen and CEO Alex Dunmow say that traditional jobs are increasingly at risk through factors such as automation.
According to the game developers, blockchain games can and are playing a vital role for people to earn a living in the digital world through play-to-earn (P2E) and move-to-earn.
The process often requires significant work, but Dunmow says many mainstream triple-A games already feature “grinding for hundreds of hours,” though the assets “provide no value for the player.”
In GameFi titles digital assets can come in the form of nonfungible tokens (NFTs); users can then take them to a marketplace and sell them for fiat currency or crypto, essentially earning a wage through gaming, argued Dunmow:
“NFTs can give you the technical ability to take ownership of a game asset out of the control of the publisher of the game.”
One of the best examples Dunmow has seen of people making a living through GameFi was a 2021 report about a community in the Philippines who turned to NFT gaming during COVID, which was now causing a shortage of workers in low-paying jobs as they could earn wages playing blockchain games instead.
“I saw the whole situation as a positive, a group of people who were likely being exploited in their low paying day jobs, have found a way to earn wages in the Metaverse.”
Dunmow and Nguyen say the negativity around NFTs and blockchain in gaming present a challenge, but through their games, they hope to “subtly educate people about the benefits of NFTs.”
The game studio has been developing a set of blockchain games under the “Supremacy World” ecosystem, which involves building, fighting and mining resources within a fictional dystopian world where factions use giant mechs to fight for territory and power.
Supremacy will eventually combine four games, a battle arena which is already out, a first-person shooter (FPS), an MMO and a real-time grand strategy (RTS) game.
Through the series of four interoperable games, the executives said they are creating an ecosystem where players have “sovereign ownership” over their digital assets and can use them in whatever way they want, explaining:
“What interoperable boils down to is being able to share digital assets between games.”
However, Nguyen noted that this interoperability also can also extend to “other game worlds, DeFi and PFP collection.”
“Supremacy will give people who own an NFT, whatever it may be, in-game assets in our world,” said Nguyen, adding that they recently were given a chance to design a custom mech skin for a user based on his Bored Apes’ Yacht Club brand NFT, noting that he can now connect his “ape” and claim his custom skin based on his NFT.
Although given the time and resources required, Dunmow acknowledges they won’t be able to custom design something for every user, but he says it shows what is possible.
Related: Illuvium co-founder shares plans for new ‘interoperable blockchain game’ model
Dunmow said that at the heart of their game, they’re still trying to build a “fun game” which he believes is vital to the industry’s survival, adding that “attracting players from outside the crypto space is crucial, especially in bear markets.”
“You make a fun game that has blockchain elements and attracts mainstream players; you are now disconnected from market forces, and you’ll be able to survive any recession.”
On Oct. 5, Ninja Syndicate announced a new deal with NFT minting and trading platform Immutable X allowing them to build on Immutable X’s layer-2 ecosystem, joining projects including Illuvium, Gods Unchained and GameStop.
[ad_2]